https://soo0100.tistory.com/1713
스크롤 뷰 만들기 와 주의사항
화면에 넘치는 데이터를 처리하는 것 중 가장 기본적인 것이 바로 스크롤 뷰이다. 스크롤 뷰에서 주의 사항은 다음과 같다. 1. 스크롤 뷰의 자식은 하나만 생성한다. 2. 스크롤 뷰 내부의 layout_hei
soo0100.tistory.com
지난 시간 살펴보았던 스크롤 뷰는 고정 데이터 혹은 간략한 데이터를 처리 하기에는 좋지만
많은 양의 데이터나 이미지 등을 나타내기에는 무리가 따른다. (메모리 릭 발생)
그렇기에 많은 양의 데이터를 다룰때는 리스트 뷰 혹은 그리드 뷰를 사용한다.
리스트 뷰나 그리드 뷰는 AdapterView 라는 추상클래스를 상속받고 어댑터 패턴을 사용해 데이터를 뷰에 표시합니다.
오늘은 바로 어댑터 뷰를 이용한 데이터 처리의 기본과정을 정리해본다. 먼저, 결과 값은 하기처럼 보여진다.
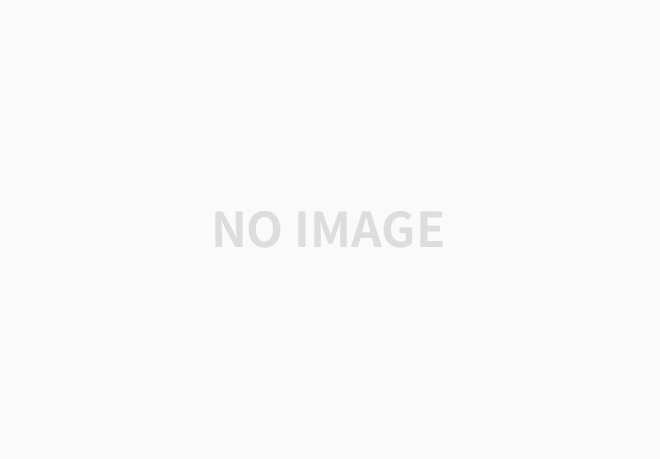
위 와 같은 리스트뷰를 만들어 본다.
1. 화면을 구성하자. (먼저 XML 로 화면을 만들어 둔다)
ListView 로 화면 전체를 커버한다. 부모객체는 어떠하든 상관없다. ListView 를 썼다는게 중요하다.
그리고 listView 를 어댑터 와 연결시키기 위해서 꼭 id 를 설정한다.
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ListView
android:id="@+id/listview"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
2. 이제, 화면에 보여질 데이터를 만들어보자.
테스트 예제이기때문에 정적인 고정데이터를 사용했다.
하지만 서버에서 가지고 온다면 더 효율성 높은 데이터가 될것이다.
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//데이터 준비완료
ArrayList<String> data = new ArrayList<>();
for (int i = 0; i < 30; i++) {
data.add("List data = " + i);
}
화면을 만들었고, 그 화면에 보여질 데이터를 만들었다.
그럼 이제 데이터를 화면에 보여질수 있도록 연결하는 과정이 필요하다. 바로 이게 어댑터의 역할이다.
#어댑터 라는 영단의 뜻 역시 다른 전기가 기계장치를 연결해주는 장치를 의미한다.
3. 데이터 와 어댑터 를 연결한다.
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//데이터 준비완료
ArrayList<String> data = new ArrayList<>();
for (int i = 0; i < 30; i++) {
data.add("List data = " + i);
}
//어댑터 (뷰와 데이터를 연결)
ArrayAdapter<String> adapter = new ArrayAdapter<String>
(this, android.R.layout.simple_list_item_1, data);
ArrayAdapter 라는 어댑터 클래스를 통해 이미지가 없는 단순한 텍스트 위주의 정보를 리스트 뷰 형태로 표현할 수 있다. 생성자 세번째 인자값에 준비한 데이터 를 넣어준다.
데이터와 어댑터의 연결이 끝났다. 그럼 이제는 뷰와 데이터를 연결하면 되겠군 ^^
4. 리스트 뷰의 setAdapter 함수를 사용해서 뷰와 어댑터를 연결한다.
ListView listView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//데이터 준비완료
ArrayList<String> data = new ArrayList<>();
for (int i = 0; i < 30; i++) {
data.add("List data = " + i);
}
//어댑터 (뷰와 데이터를 연결)
ArrayAdapter<String> adapter = new ArrayAdapter<String>
(this, android.R.layout.simple_list_item_1, data);
// 뷰에 어댑터를 설정.
listView = findViewById(R.id.listview);
listView.setAdapter(adapter);
그림으로 표현하자면 하기와 같다.
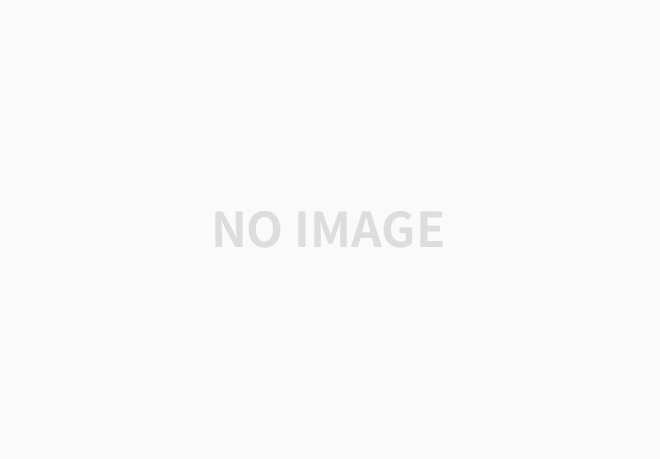
마지막으로, 해당 리스트가 잘 동작하는 지 확인차 토스트 메시지를 띄운다.
ListView listView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//데이터 준비완료
ArrayList<String> data = new ArrayList<>();
for (int i = 0; i < 30; i++) {
data.add("List data = " + i);
}
//어댑터 (뷰와 데이터를 연결)
ArrayAdapter<String> adapter = new ArrayAdapter<String>
(this, android.R.layout.simple_list_item_1, data);
// 뷰에 어댑터를 설정.
listView = findViewById(R.id.listview);
listView.setAdapter(adapter);
// 클릭 이벤트 구현
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) {
Toast.makeText(MainActivity.this, i + " 번째 데이터",
Toast.LENGTH_LONG).show();
}
});
}
이렇게 기본적인 텍스트 만 표현하는 리스트 뷰인 경우에는
ArrayAdapter 를 사용하면 된다. 하지만 우리가 흔히보는 이미지, 텍스트, 영상 등등이 섞여 있는 복잡한 리스트 형식을 구현하기 위해서는 BaseAdapter 라는 개발자가 구현하는 Adapter 가 필수 이다.
BaseAdpter 정리는 다음 포스팅에서 진행하겠다.
감사합니다.
https://soo0100.tistory.com/1731
커스텀 어댑터 뷰를 활용한 리스트뷰 구현하기-1
https://soo0100.tistory.com/1716 안드로이드 어댑터 뷰 기초(리스트 뷰 만들기) https://soo0100.tistory.com/1713 스크롤 뷰 만들기 와 주의사항 화면에 넘치는 데이터를 처리하는 것 중 가장 기본적인 것이 바..
soo0100.tistory.com
https://soo0100.tistory.com/1732
커스텀 어댑터 뷰를 활용한 리스트뷰 구현하기-2
https://soo0100.tistory.com/1731 커스텀 어댑터 뷰를 활용한 리스트뷰 구현하기-1 https://soo0100.tistory.com/1716 안드로이드 어댑터 뷰 기초(리스트 뷰 만들기) https://soo0100.tistory.com/1713 스크롤 뷰..
soo0100.tistory.com
'앱 만들기 > 안드로이드 study' 카테고리의 다른 글
Getter,Setter 함수란 (2) | 2022.02.08 |
---|---|
모델 클래스 란? (6) | 2022.02.07 |
android:exported 에러 수정하기 (4) | 2022.01.28 |
스크롤 뷰 만들기 와 주의사항 (4) | 2022.01.27 |
HTTP 2.0 OkHttpClient 로 네트워크 구현. (2) | 2022.01.23 |
댓글