https://soo0100.tistory.com/1716
안드로이드 어댑터 뷰 기초(리스트 뷰 만들기)
https://soo0100.tistory.com/1713 스크롤 뷰 만들기 와 주의사항 화면에 넘치는 데이터를 처리하는 것 중 가장 기본적인 것이 바로 스크롤 뷰이다. 스크롤 뷰에서 주의 사항은 다음과 같다. 1. 스크롤 뷰
soo0100.tistory.com
이미지와 텍스트 정보 등이 혼용되는 리스트 뷰 를 구현하기 위해서는
BaseAdpater 클래스를 상속받아 개발자가 직접 구현하는 커스텀 어댑터를 생성해야 한다.
우리가 주로 앱에서 많이 보는 리 스튜 뷰의 타입이 바로 커스텀 어댑터 뷰이다.
구현 결과물은 하기와 같다.
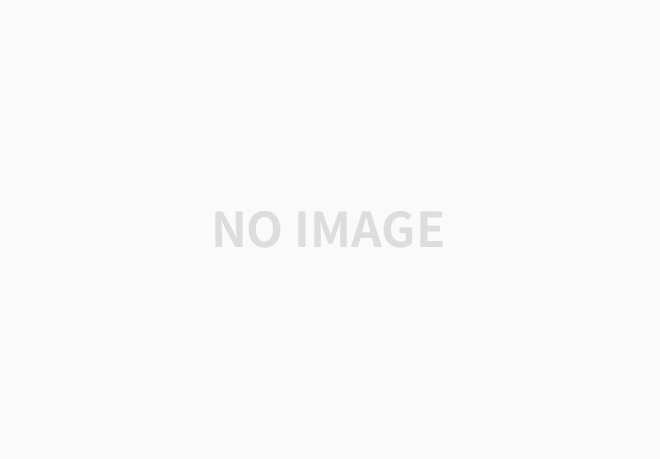
위 데이터가 로컬이 아닌 서버에서 받게 된다면, 그럴듯한 앱이 되는 것이다.
구현을 해보자.
1. 화면을 구성하자. (먼저 XML로 화면을 만들어 둔다)
ListView로 화면 전체를 커버한다. 부모 객체는 어떠하든 상관없다. ListView를 썼다는 게 중요하다.
그리고 listView 를 어댑터와 연결시키기 위해서 꼭 id를 설정한다.
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ListView
android:id="@+id/listview"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
2. 이제, 화면에 보여질 데이터를 만들어보자.
테스트 예제 이기때문에 로컬에서 만드는 고정 데이터를 사용했다.
하지만 서버에서 가지고 온다면 위에서 이야기한 것처럼 그럴듯한 앱이 될 것이다.
고정 데이터를 체계적으로 관리하기 위해서, 앞 시간에 살펴보았던 모델 클래스를 활용하여 데이터를 저장한다.
https://soo0100.tistory.com/1723
모델 클래스 란?
모델 클래스란, 실제의 형태를 클래스 형태로 만드는 것을 말한다. 즉, 클래스로 만들어서 데이터를 저장하고 가져오는 역할을 하게 된다. 예를 들어 날씨를 클래스로 만든다면, 일반적으로 지
soo0100.tistory.com
날씨 정보를 저장하는 모델클래스의 정의는 하기와 같다.
필드 값과 Getter,Setter 함수 그리고, 디버깅을 위한 toString() 함수가 존재한다.
https://soo0100.tistory.com/1724
Getter,Setter 함수란
https://soo0100.tistory.com/1723 모델 클래스 란? 모델 클래스란, 실제의 형태를 클래스 형태로 만드는 것을 말한다. 즉, 클래스로 만들어서 데이터를 저장하고 가져오는 역할을 하게 된다. 예를 들어 날
soo0100.tistory.com
//모델 클래스
public class Weather {
private String city;
private String temp;
private String weather;
public Weather(String city, String temp, String weather) {
this.city = city;
this.temp = temp;
this.weather = weather;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getTemp() {
return temp;
}
public void setTemp(String temp) {
this.temp = temp;
}
public String getWeather() {
return weather;
}
public void setWeather(String weather) {
this.weather = weather;
}
@Override
public String toString() {
return "Weather{" +
"city='" + city + '\'' +
", temp='" + temp + '\'' +
", weather='" + weather + '\'' +
'}';
}
}
데이터를 위한 모델클래스를 정의 했으니,
Listview 에 담을 데이터를 ArrayList<Weather> 형으로 만들어 본다.
public class MainActivity extends AppCompatActivity {
ListView listView;
ArrayList<Weather>data = new ArrayList<>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
///임시 데이터 만들기.
data.add(new Weather("서울","8도","맑음"));
data.add(new Weather("인천","5도","맑음"));
data.add(new Weather("수원","7도","맑음"));
data.add(new Weather("오산","8도","맑음"));
data.add(new Weather("대전","7도","맑음"));
data.add(new Weather("전주","6도","맑음"));
data.add(new Weather("광주","5도","맑음"));
data.add(new Weather("대구","8도","맑음"));
data.add(new Weather("부산","9도","맑음"));
data.add(new Weather("제주도","10도","맑음"));
}
}
자 여기까지 하면, 먼저 화면을 만들었고, 그 화면에 보여질 데이터까지 모두 완료가 된것이다.
그럼 이제 데이터를 화면에 보여질 수 있도록 연결하는 과정이 필요하다. 바로 이것이 어댑터의 역할이다.
이번 시간은 기본 어댑터뷰가 아니라 개발자가 만드는 커스텀 어댑터 뷰이다.
포스팅이 길어지는 관계로 커스텀 어댑터 뷰 구현은 다음 글에서 이어진다.
감사합니다.
https://soo0100.tistory.com/1732
커스텀 어댑터 뷰를 활용한 리스트뷰 구현하기-2
https://soo0100.tistory.com/1731 커스텀 어댑터 뷰를 활용한 리스트뷰 구현하기-1 https://soo0100.tistory.com/1716 안드로이드 어댑터 뷰 기초(리스트 뷰 만들기) https://soo0100.tistory.com/1713 스크롤 뷰..
soo0100.tistory.com
'앱 만들기 > 안드로이드 study' 카테고리의 다른 글
자바 예외처리가 필요한 이유 (4) | 2022.03.12 |
---|---|
커스텀 어댑터 뷰를 활용한 리스트뷰 구현하기-2 (4) | 2022.02.15 |
안드로이드 스튜디오 implement 함수 구현하는 방법 (4) | 2022.02.11 |
생성자 손쉽게 만들기 (2) | 2022.02.10 |
Getter,Setter 함수란 (2) | 2022.02.08 |
댓글